Advertising
Overview
GamePush allows you to implement the display of advertisements or rewarded videos in the game. Advertising in GamePush is handled using the Ads Manager gp.ads
. The manager manages banners on the game page:
- Ad display;
- Automatic update timer management;
- Advertising show frequency.
Supported platforms
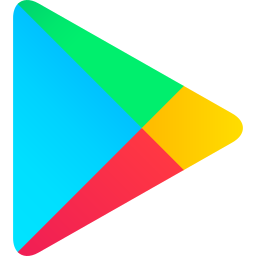
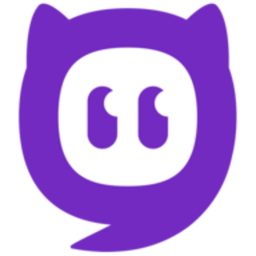
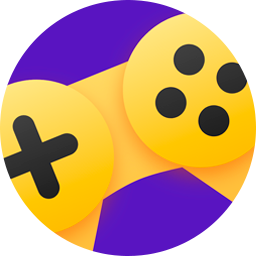
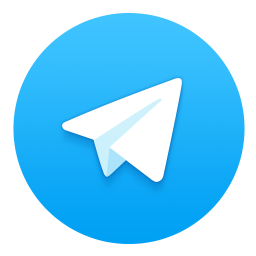
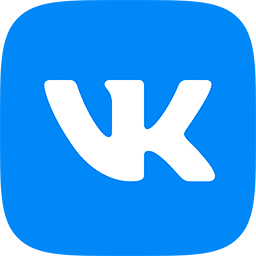
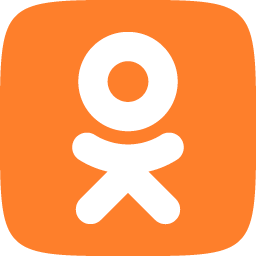
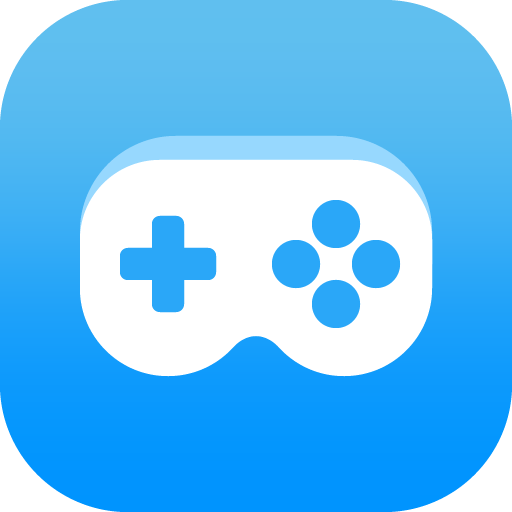
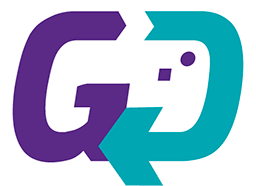
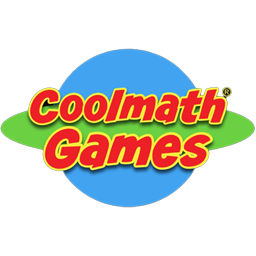
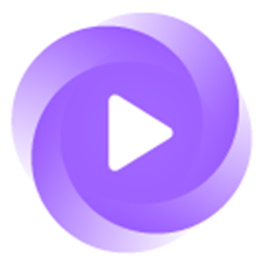
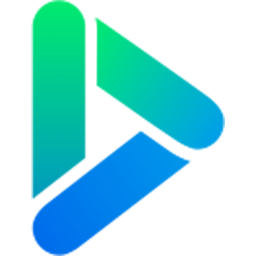
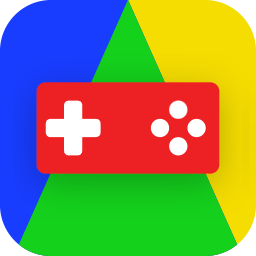
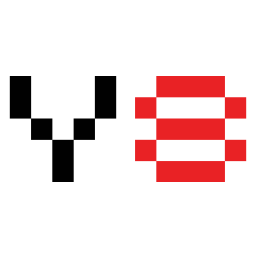
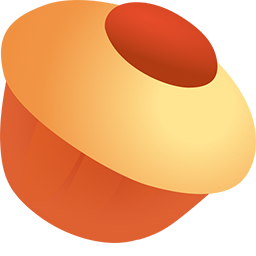
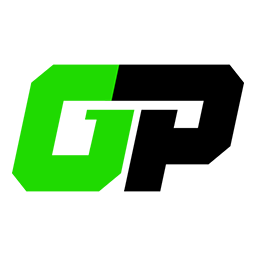
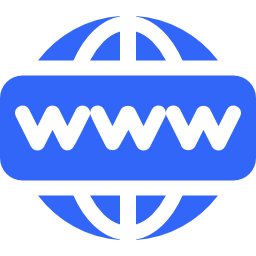
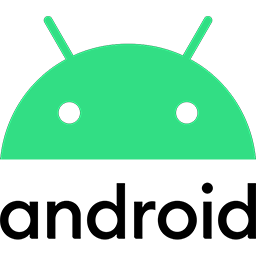
Platforms without support
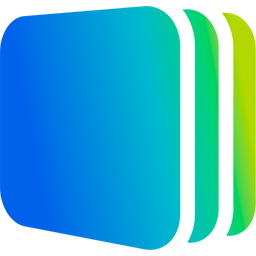
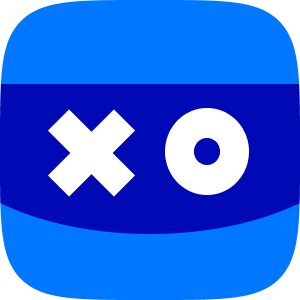
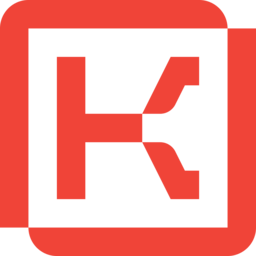
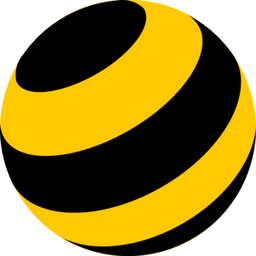
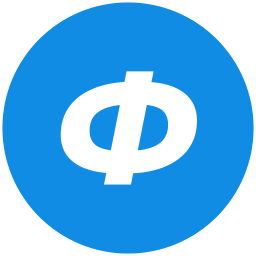
Additional Sections
🗃️ Third-Party Advertising Providers
6 items
Ad Manager
FREEThe ad manager rules over the banners on the page. Its responsibility consists in displaying ads, managing auto-refresh and ads frequency timers.
Available ad manager properties:
- JavaScript
- Unity
// Is AdBlock enabled
gp.ads.isAdblockEnabled;
// Is the banner available
gp.ads.isStickyAvailable;
gp.ads.isFullscreenAvailable;
gp.ads.isRewardedAvailable;
gp.ads.isPreloaderAvailable;
// Is the ads playing now
gp.ads.isStickyPlaying;
gp.ads.isFullscreenPlaying;
gp.ads.isRewardedPlaying;
gp.ads.isPreloaderPlaying;
// Check enabled advertising overlays
// Countdown overlay before displaying fullscreen ads is enabled
gp.ads.isCountdownOverlayEnabled;
// Overlay is enabled for failed display of rewarded video
gp.ads.isRewardedFailedOverlayEnabled;
// Can fullscreen ads be shown on the platform before gameplay starts
gp.ads.canShowFullscreenBeforeGamePlay;
// Is AdBlock enabled
GP_Ads.IsAdblockEnabled();
// Is ads available
GP_Ads.IsFullscreenAvailable();
GP_Ads.IsPreloaderAvailable();
GP_Ads.IsRewardedAvailable();
GP_Ads.IsStickyAvailable();
// Is the ads playing now
GP_Ads.IsFullscreenPlaying();
GP_Ads.IsPreloaderPlaying();
GP_Ads.IsRewardPlaying();
GP_Ads.IsStickyPlaying();
/// Check enabled advertising overlays
// Countdown overlay before displaying fullscreen ads is enabled
GP_Ads.IsCountdownOverlayEnabled();
// Overlay is enabled for failed display of rewarded video
GP_Ads.IsRewardedFailedOverlayEnabled();
// Can fullscreen ads be shown on the platform before gameplay starts
GP_Ads.CanShowFullscreenBeforeGamePlay();
You can subscribe for the following basic events:
- JavaScript
- Unity
// Ads show started
gp.ads.on('start', () => {});
// Ads show ended
gp.ads.on('close', (success) => {});
private void OnEnable()
{
GP_Ads.OnAdsStart += OnAdsStart;
GP_Ads.OnAdsClose += OnAdsClose;
}
private void OnDisable()
{
GP_Ads.OnAdsStart -= OnAdsStart;
GP_Ads.OnAdsClose -= OnAdsClose;
}
private void OnAdsStart(){
// When ads started
}
private void OnAdsClose(bool success){
// When ads ended
}
Fullscreen
FREEFullscreen banner or interstitial is pop-up, often fullscreen skippable (sometimes only after a few seconds) advertising. Usually it is shown in transition between levels. Its displaying is prohibited during the gameplay on many platforms. It is allowed to show only in pause between game sessions.
It is also prohibited during the navigation by the VK Games platform. So, we will consider this a bad practice on other platforms too.
Call example:
- JavaScript
- Unity
// Show fullscreen, returns a promise
gp.ads.showFullscreen();
// Show fullscreen with a countdown overlay before displaying the advertisement
gp.ads.showFullscreen({ showCountdownOverlay: true });
// Showing started
gp.ads.on('fullscreen:start', () => {});
// Showing ended
gp.ads.on('fullscreen:close', (success) => {});
// Show fullscreen
public void ShowFullscreen() => GP_Ads.ShowFullscreen(OnFullscreenStart, OnFullscreenClose);
// Showing started
private void OnFullscreenStart() => Debug.Log("ON FULLSCREEN START");
// Showing ended
private void OnFullscreenClose(bool success) => Debug.Log("ON FULLSCREEN CLOSE");
Preloader
FREEPleloader is a banner that appears when loading the game. On many platforms, it is implemented through Fullscreen, but is not tied to its timers (except for Yandex.Games).
Displaying is allowed only before the start of the game.
Call example:
- JavaScript
- Unity
// Show preloader, returns a promise
gp.ads.showPreloader();
// Showing started
gp.ads.on('preloader:start', () => {});
// Showing ended
gp.ads.on('preloader:close', (success) => {});
// Show preloader
public void ShowPreloader() => GP_Ads.ShowPreloader(OnPreloaderStart, OnPreloaderClose);
// Showing started
private void OnPreloaderStart() => Debug.Log("ON PRELOADER: START");
// Showing ended
private void OnPreloaderClose(bool success) => Debug.Log("ON PRELOADER: CLOSE");
Rewarded Video
FREERewarded Video is a non-skippable video ad that aims to give the player a reward for watching. It is forbidden to show without a reward.
Call example:
- JavaScript
- Unity
// Show rewarded video, returns a promise
gp.ads.showRewardedVideo();
// Show rewarded video with an option to display an overlay
// in case of an unsuccessful display of the rewarded video
gp.ads.showRewardedVideo({ showFailedOverlay: true });
// Asynchronously
const success = await gp.ads.showRewardedVideo();
if (success) {
gp.player.add('gold', 5000);
}
// Showing started
gp.ads.on('rewarded:start', () => {});
// Showing ended
gp.ads.on('rewarded:close', (success) => {});
// Reward is received
gp.ads.on('rewarded:reward', () => {});
// Show rewarded video
public void ShowRewarded() => GP_Ads.ShowRewarded("COINS", OnRewardedReward, OnRewardedStart, OnRewardedClose);
// Showing started
private void OnRewardedStart() => Debug.Log("ON REWARDED: START");
// Reward is received
private void OnRewardedReward(string value)
{
if (value == "COINS")
Debug.Log("ON REWARDED: +150 COINS");
if (value == "GEMS")
Debug.Log("ON REWARDED: +5 GEMS");
}
// Showing ended
private void OnRewardedClose(bool success) => Debug.Log("ON REWARDED: CLOSE");
Sticky Banner
FREESticky banner is a fixed bottom banner. It takes ~50-100px (110px VK Direct Games). The banner must not cover the play region.
In the panel, you can customize the auto-refresh frequency. The banner will be updated according to the specified frequency immediately of the start.
Call example:
- JavaScript
- Unity
// Show the sticky banner, then it will auto-update itself
gp.ads.showSticky();
// Refresh the sticky banner, forced refreshing
gp.ads.refreshSticky();
// Close the sticky banner
gp.ads.closeSticky();
// Open the banner
gp.ads.on('sticky:start', () => {});
// The banner appears on the screen
gp.ads.on('sticky:render', () => {});
// The banner updated
gp.ads.on('sticky:refresh', () => {});
// Close the banner
gp.ads.on('sticky:close', () => {});
// Show the sticky banner, then it will auto-update itself
public void ShowSticky() => GP_Ads.ShowSticky();
// Refresh the sticky banner, forced refreshing
public void RefreshSticky() => GP_Ads.RefreshSticky();
// Close the sticky banner
public void CloseSticky() => GP_Ads.CloseSticky();
// Open the banner
private void OnStickyStart() => Debug.Log("ON STICKY: START");
// Close the banner
private void OnStickyClose() => Debug.Log("ON STICKY: CLOSE");
// The banner appears on the screen
private void OnStickyRender() => Debug.Log("ON STICKY: RENDER");
// The banner updated
private void OnStickyRefresh() => Debug.Log("ON STICKY: REFRESH");
Supported platforms
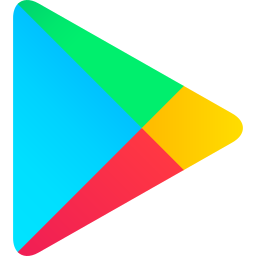
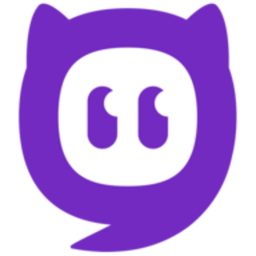
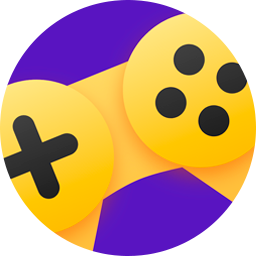
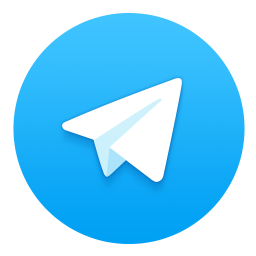
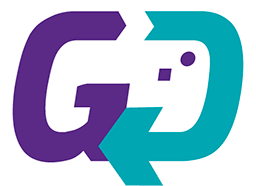
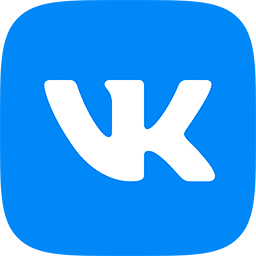
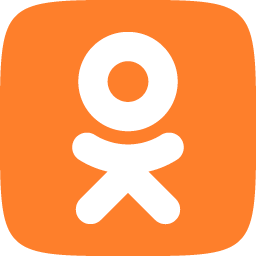
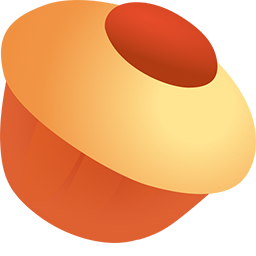
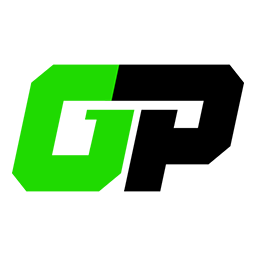
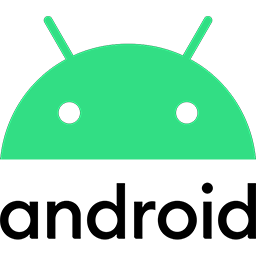
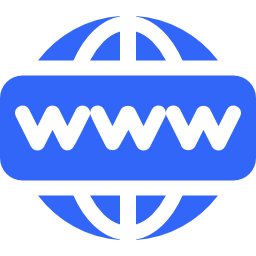
Platforms without support
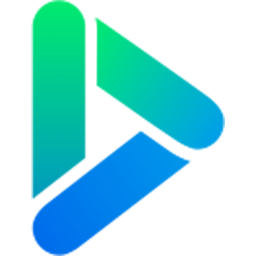
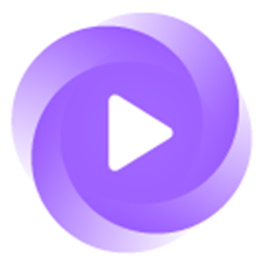
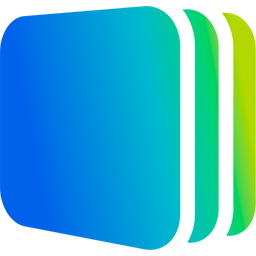
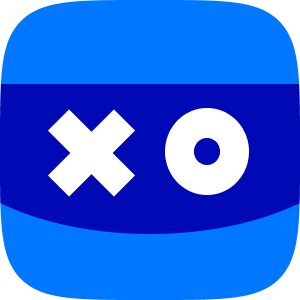
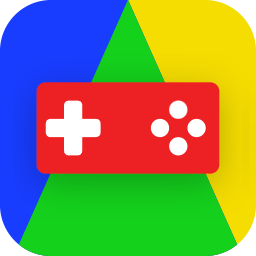
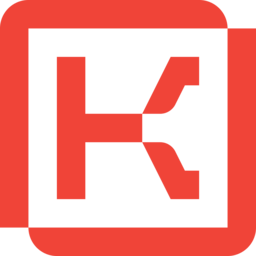
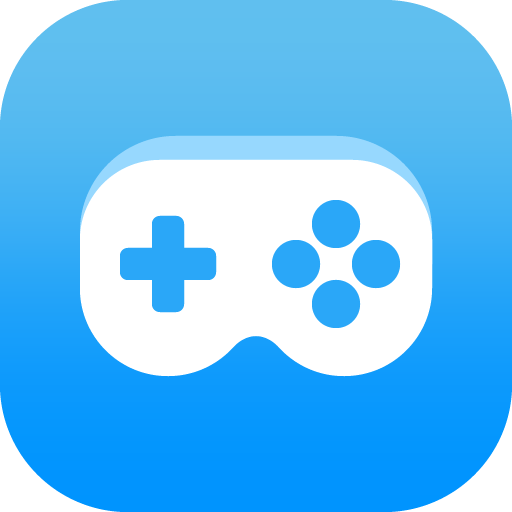
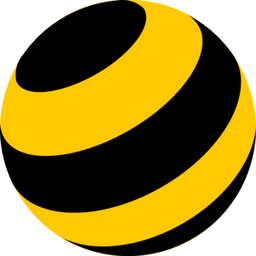
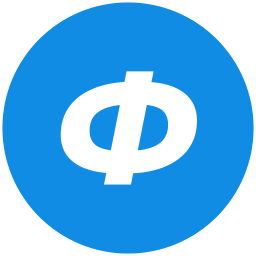
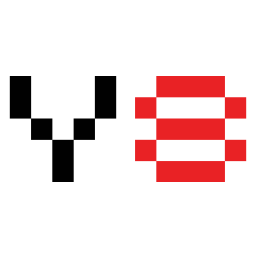
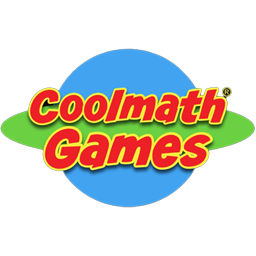
Stay in Touch
Other documents of this chapter available Here. To get started, welcome to the Tutorials chapter.
GamePush Community Telegram
: @gs_community.
For your suggestions e-mail
: official@gamepush.com
We Wish you Success!