Subscriptions
Process players' recurring payments.
Overview
The service allows your players to buy subscriptions. For example, a player can buy a VIP access that requires a monthly renewal. Payments for goods and payments for subscriptions differ in the frequency of debiting funds from the user's account. One-time payments are debited once per purchase, while subscription payments are debited at regular intervals.
- Subscriptions are best suited for VIP accounts and sales of bonus.
- Subscriptions can also be used to access regularly updated content, such as new levels or characters.
Thus, a subscription is a product that has such fields as:
- Subscription ID - boolean field (true = subscription / false = product);
- Subscription period - 7 and 30, 90 days;
- Trial period - 0, 3, 7 and 30 days;
Field | Type | Description | Example |
---|---|---|---|
isSubscription | boolean | Purchase is a subscription | default false |
period | number | Subscription duration in days | 7 , 30 , 90 |
trialPeriod | number | The duration of the trial period in days (during this time, the user can cancel the subscription without debiting funds) | 0 , 3 , 7 , 30 |
In the SDK, you have access to information on purchased player subscriptions, they expand the standard purchase.
Field | Type | Description | Example |
---|---|---|---|
subscribed | boolean | Checking if a purchase subscription is active | true |
expiredAt | string | Subscription end date, format ISO 8601 UTC | 2022-12-01T04:52:26+0000 |
Supported platforms
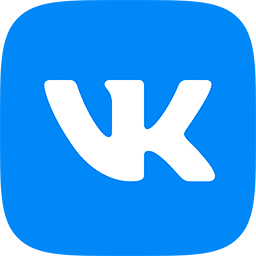
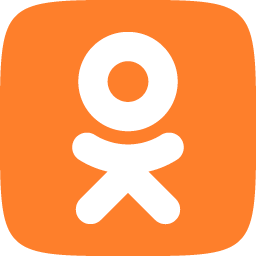
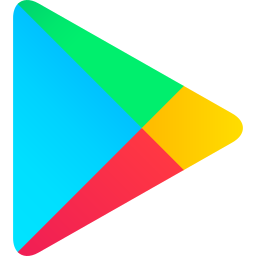
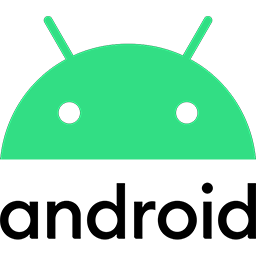
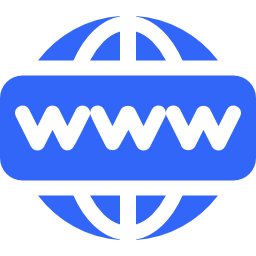
Platforms without support
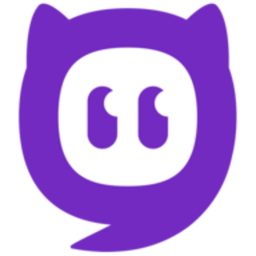
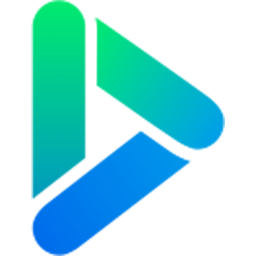
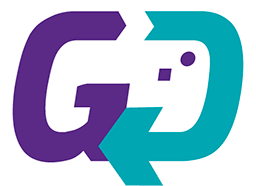
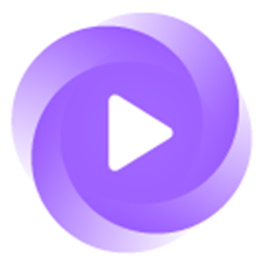
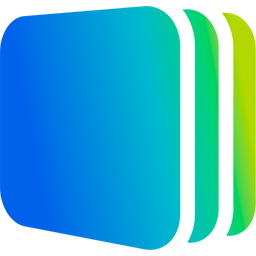
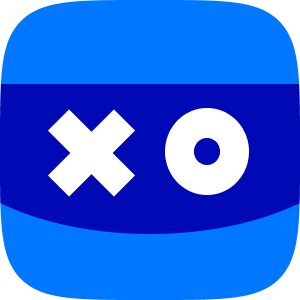
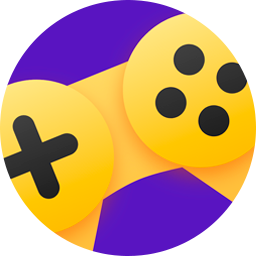
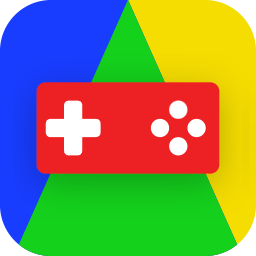
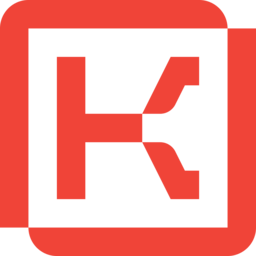
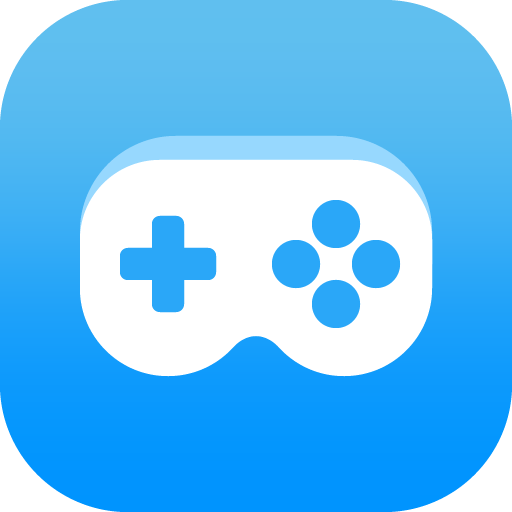
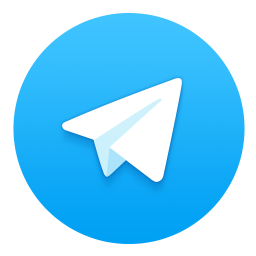
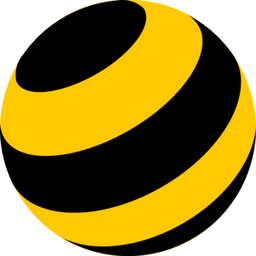
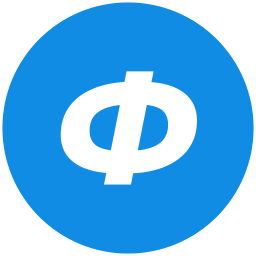
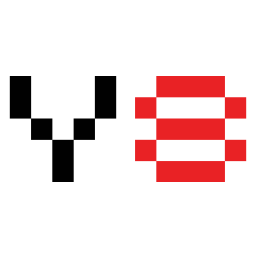
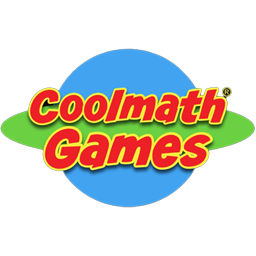
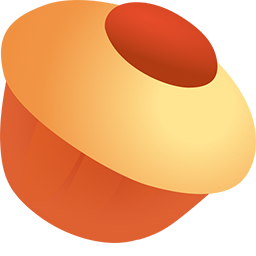
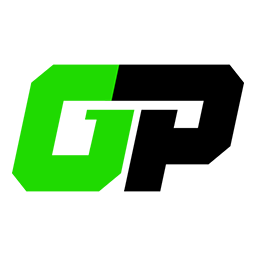
Supported platforms (one-time subscriptions)
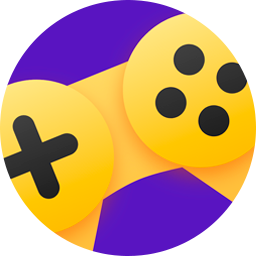
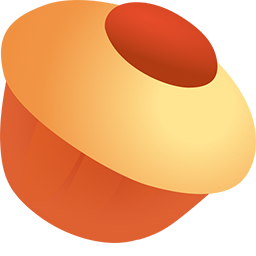
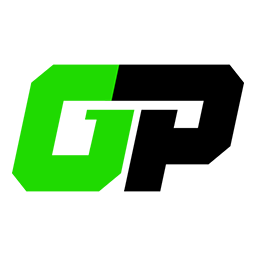
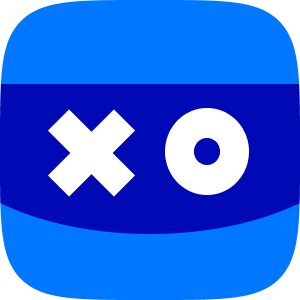
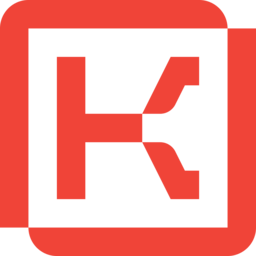
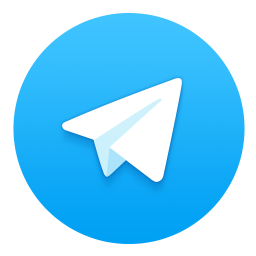
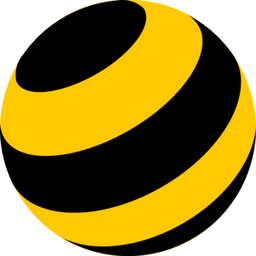
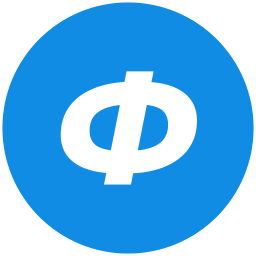
Platforms without support
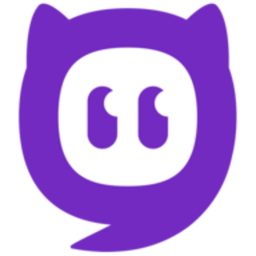
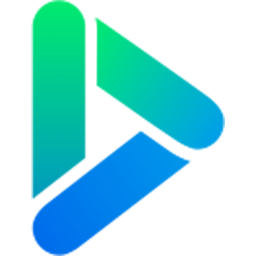
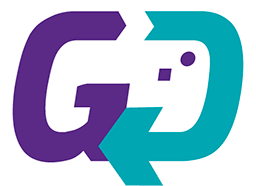
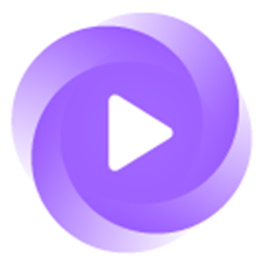
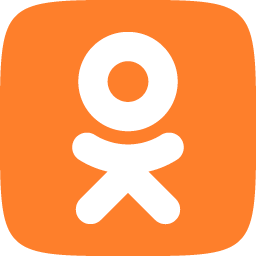
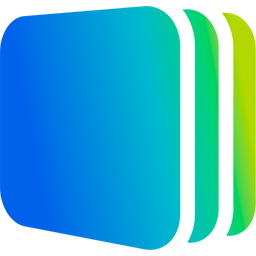
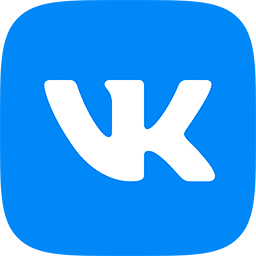
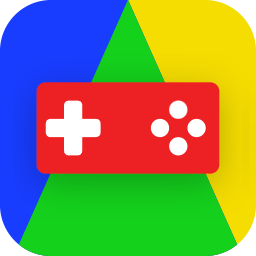
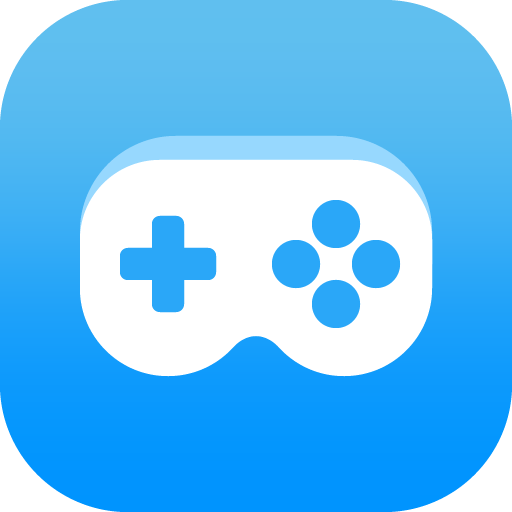
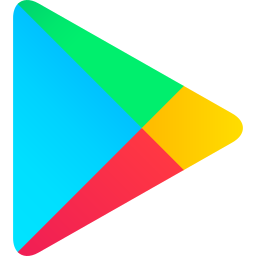
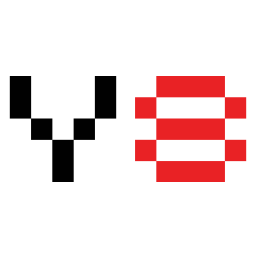
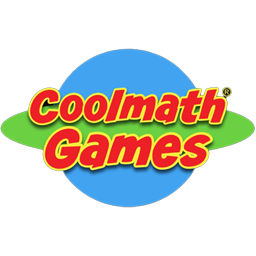
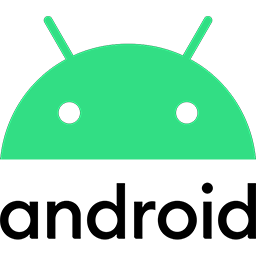
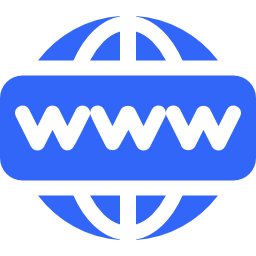
Use the isSubscriptionsAvailable
property to check for on-platofrm subscription support in the SDK.
- JavaScript
- Unity
// Check for subscription support on the platform
if (gp.payments.isSubscriptionsAvailable) {
// you can subscribe
}
// Check for subscription support on the platform
if (GP_Payments.IsSubscriptionsAvailable()) {
// you can subscribe
}
Subscription period and trial period
The period is specified in days. For the test period are available: 0
, 3
, 7
and 30
days. The trial period is the period when money is not withdrawn from the player and he can cancel the subscription free of charge. At the end of the test period, the site writes off the funds.
The subscription period can be specified in 7
, 30
and 90
days. The subscription will automatically renew at the end of the period, unless cancelled.
Suggested periods in 7
, 30
and 90
days are due to a single implementation of subscriptions on all sites. Some sites allow only such values.
Subscriptions
Setting up subscriptions on the platforms
Platforms setup are described in payments section, nothing else is required from the technical side. Pay attention to the features of working with platforms.
Adding a subscription
To enable a subscription, you need to select a purchase and transfer it to the subscription status using the "Enable Subscription" toggle switch.
Now you can subscribe to this product.
If you buy a subscription through the purchase method purchase
, then it will be issued as a subscription without automatic renewal and will be used up after the period specified in the purchase
Subscribing
+1-3 RequestTo subscribe, use the subscribe
method.
- JavaScript
- Unity
// by ID
gp.payments.subscribe({ id: 12 });
// by Tag
gp.payments.subscribe({ tag: 'VIP' });
// by ID
GP_Payments.Subscribe("12");
// by Tag
GP_Payments.Subscribe("VIP");
The result of the call can be found out by subscribing to events subscribe
.
- JavaScript
- Unity
// The player has subscribed
gp.payments.on('subscribe', ({ product, purchase }) => {});
// Subscription not registered
gp.payments.on('error:subscribe', (error) => {});
public void Subscribe() => GP_Payments.Subscribe("VIP", OnSubscribeSuccess, OnSubscribeError);
// The player has subscribed
private void OnSubscribeSuccess(string idOrTag) => Debug.Log("SUBSCRIBE: SUCCESS: " + idOrTag);
// Subscription not registered
private void OnSubscribeError() => Debug.Log("SUBSCRIBE: ERROR");
When used asynchronously:
- JavaScript
- Unity
const result = await gp.payments.subscribe({ id: 17541 });
/**
* Product data
* @type {Product}
*/
result.product;
/**
* Purchase data
* @type {PlayerPurchase}
*/
result.purchase;
Not implemented
Cancel subscription
+0-1 RequestThe player should be able to unsubscribe. To unsubscribe, use the method unsubscribe
.
Canceling a subscription does not mean deleting a player's purchase. The purchase will be valid until the end of the period.
- JavaScript
- Unity
// by ID
gp.payments.unsubscribe({ id: 12 });
// by Tag
gp.payments.unsubscribe({ tag: 'VIP' });
// by ID
GP_Payments.Unsubscribe("12");
// by Tag
GP_Payments.Unsubscribe("VIP");
The result of the call can be found out by subscribing to events unsubscribe
.
- JavaScript
- Unity
// Player unsubscribed
gp.payments.on('unsubscribe', ({ product, purchase }) => {});
// Failed to unsubscribe
gp.payments.on('error:unsubscribe', (error) => {});
public void Unsubscribe() => GP_Payments.Unsubscribe("VIP", OnUnsubscribeSuccess, OnUnsubscribeError);
// Player unsubscribed
private void OnUnsubscribeSuccess(string idOrTag) => Debug.Log("UNSUBSCRIBE: SUCCESS: " + idOrTag);
// Failed to unsubscribe
private void OnUnsubscribeError() => Debug.Log("UNSUBSCRIBE: ERROR");
When used asynchronously:
- JavaScript
- Unity
const result = await gp.payments.unsubscribe({ id: 17541 });
/**
* Product data
* @type {Product}
*/
result.product;
/**
* Purchase data
* @type {PlayerPurchase}
*/
result.purchase;
Not implemented
Subscription renewal
+0-3 RequestAfter canceling a subscription, while it is still valid, it is possible to renew the subscription. Call the subscription method again to resume the subscription.subscribe for the same item.
Subscription testing
We have prepared for you a convenient tool for testing subscriptions without the need to test directly on the sites.
Test subscriptions automatically renew at the end of their term. To test the subscription expiration, you can set the subscription expiration date to the previous day in the control panel. Every 10 minutes we delete expired subscriptions.
After cancellation, the subscription will still be valid until the expiration date, then it will be deleted. You can renew your subscription while it's still active.
Interface to change the subscription expiration time:
Features of working with platforms
One-time subscriptions
There is no subscription functionality on Yandex.Games, VK Play and Kongregate, but we have provided a unified work logic. It is possible to subscribe to a subscription that will not be renewed and will be deleted after the end of the subscription period. This will work like a one-time purchase, but will automatically consume when it expires.
OK Games
Before using subscriptions, you must submit a request to technical support indicating detailed information about the products you need to subscribe to. In the product ID, specify the product ID in the GamePush admin panel.
Do not delete published subscriptions or change their period information. The data is stored on the Odnoklassniki side. First, make changes in Odnoklassniki.
Implementation examples
Checking the goods. There may be a situation where you need to check that the product is a subscription. And depending on this check - buy a product, or subscribe to it, if it isSubscription
. The implementation of such a check is shown below:
- JavaScript
- Unity
if (product.isSubscription) {
gp.payments.subscribe({ id: product.id });
} else {
gp.payments.purchase({ id: product.id });
}
Not implemented
Purchase. An example of explicitly buying a subscription by id
:
- JavaScript
- Unity
gp.payments.subscribe({ id: 12 });
GP_Payments.Subscribe("12");
Subscription period fields. An example of using period fields shows how you can display information about the balance of a trial period. And also inform the player about the terms of the subscription.
$form.setText(`Free first ${product.trialPeriod} days`);
$form.setText(`Then ${product.price} ${product.currencySymbol} every ${product.period} days`);
User notice. An example of notifying the user about the approaching end of subscription event:
const purchase = gp.payments.getPurchase('VIP');
const timeBetween = new Date(purchase.expiredAt).getTime() - new Date(gs.serverTime).getTime();
const daysBetween = timeBetween / (1000 * 3600 * 24);
if (daysBetween <= 3 && !purchase.subscribed) {
alert('You have 3 days of subscription left, you have not renewed it');
}
Stay in Touch
Other documents of this chapter available Here. To get started, welcome to the Tutorials chapter.
GamePush Community Telegram
: @gs_community.
For your suggestions e-mail
: [email protected]
We Wish you Success!