Payments
Process players' one-time payments.
Supported Platforms
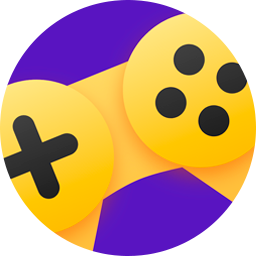
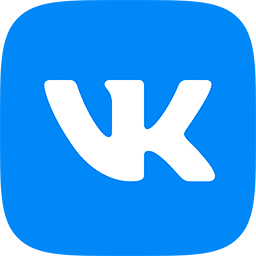
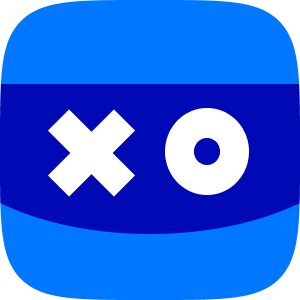
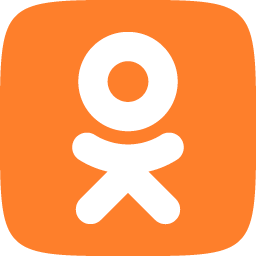
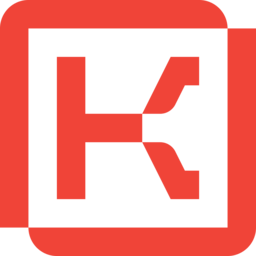
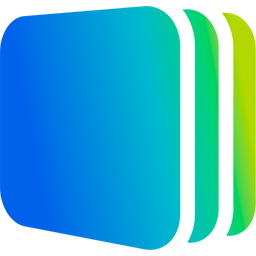
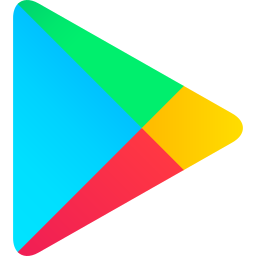
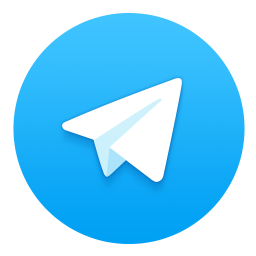
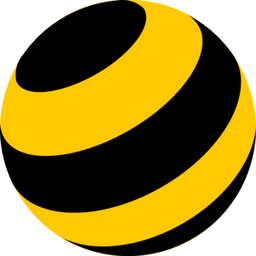
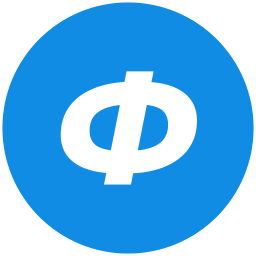
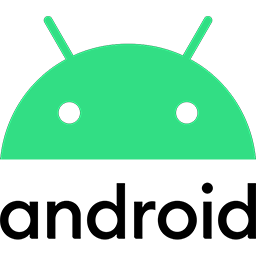
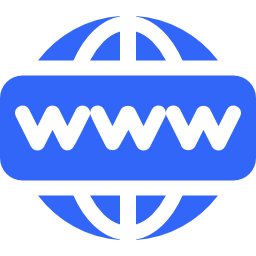
Platforms without support
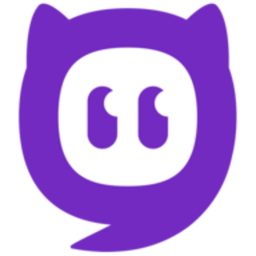
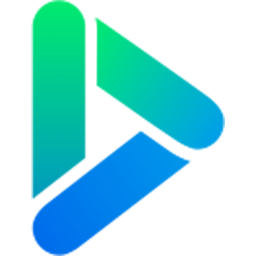
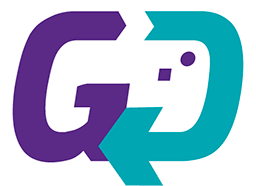
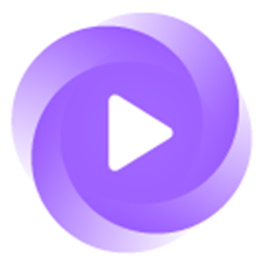
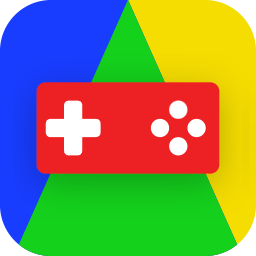
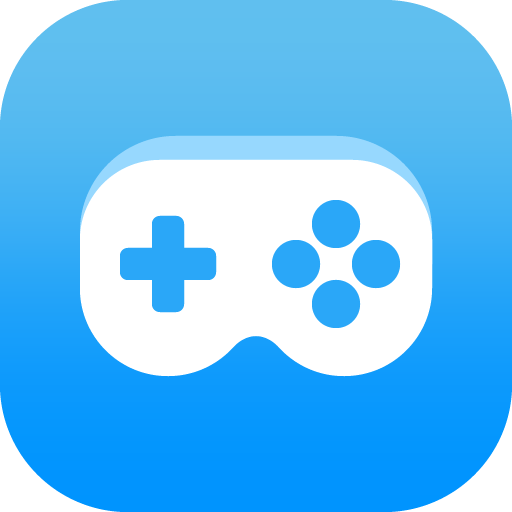
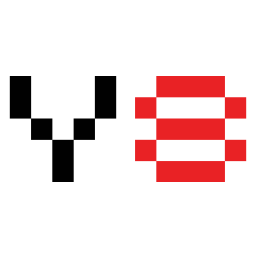
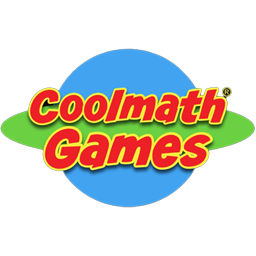
To check for purchase support on a platform in the SDK, use the gp.payments.isAvailable
property.
- JavaScript
- Unity
// Checking for purchase support on a platform
if (gp.payments.isAvailable) {
// You can make purchases
}
// Check payments on paltform
if (GP_Payments.IsPaymentsAvailable()) {
// can show / purchase products
}
Payment Rules
Stick to payments rules.
There are two types of payments: one-time and permanent.
Example of a one-time purchase:
- Buy 1000 gold
- Buy chest
- Restore Lives
One-time purchases must be consumed immediately.
An example of a permanent purchase:
- Disable ads
- VIP status
- Increase x5 reward per level
Constant purchases do not need to be consumed. As soon as the purchase is paid, it will always be visible to the user, until it is used up.
One-time purchase rules
- JavaScript
- Unity
// 1. Make purchase
await gp.payments.purchase({ tag: 'GOLD_1000' });
// 2. Give a reward
gp.player.add('gold', 1000);
// 3. Save player progess on server
await gp.player.sync();
// 4. Consume purchase
await gp.payments.consume({ tag: 'GOLD_1000' });
// 1. Make purchase
GP_Payments.Purchase("EXTRA_GOLD");
// 2. Give a reward
GP_Player.Add('gold', 1000);
// 3. Save player progess on server
GP_Player.Sync();
// 4. Consume purchase
GP_Payments.Consume("EXTRA_GOLD");
Permanent purchase rules
- JavaScript
- Unity
// 1. Make purchase
await gp.payments.purchase({ tag: 'VIP' });
// 2. Checking for purchase availability when needed
if (gp.payments.has('VIP')) {
// do something with VIP status
}
// 1. Make purchase
GP_Payments.Purchase('VIP', OnPurchaseSuccess, OnPurchaseError);
private void OnPurchaseSuccess(string productIdOrTag){
if(productIdOrTag == 'VIP')
GP_Player.Set('VIP', true);
}
private void OnPurchaseError() => Debug.Log("PURCHASE: ERROR");
// 2. Checking for purchase availability when needed
if (GP_Player.GetBool('VIP')) {
/// do something with VIP status
}
Purchase product
+1-3 RequestTo purchase product, you need to pass an ID or Tag of product.
- JavaScript
- Unity
// Purchase by id
gp.payments.purchase({ id: 17541 });
// Purchase by tag
gp.payments.purchase({ tag: 'GOLD_1000' });
// Purchase by id
GP_Payments.Purchase("15234");
// Purchase by tag
GP_Payments.Purchase("EXTRA_GOLD");
Purchase product events
- JavaScript
- Unity
// Purchase success
gp.payments.on('purchase', ({ product, purchase }) => {});
/**
* Error if purchase failed
* @type {
* 'player_not_found' |
* 'empty_id_or_tag' |
* 'product_not_found' |
* 'purchases_not_alloved_on_platform' |
* undefined
* }
*/
gp.payments.on('error:purchase', (error) => {});
public void Purchase() => GP_Payments.Purchase("EXTRA_GOLD", OnPurchaseSuccess, OnPurchaseError);
// Purchase success
private void OnPurchaseSuccess(string productIdOrTag) => Debug.Log("PURCHASE: SUCCESS: " + productIdOrTag);
// Error if purchase failed
private void OnPurchaseError() => Debug.Log("PURCHASE: ERROR");
Purchase with promises
- JavaScript
- Unity
const result = await gp.payments.purchase({ id: 17541 });
/**
* Product data
* @type {Product}
*/
result.product;
/**
* Purchase data
* @type {PlayerPurchase}
*/
result.purchase;
Not implemented
Consuming purchases
+1 RequestTo use a purchase, you need to pass in a product ID or tag.
- JavaScript
- Unity
// Spending by ID
gp.payments.consume({ id: 17541 });
// Spending by Tag
gp.payments.consume({ tag: 'GOLD_1000' });
// Spending by ID
GP_Payments.Consume("15234");
// Spending by Tag
GP_Payments.Consume("EXTRA_GOLD");
Purchase spend events
- JavaScript
- Unity
// Successfully used
gp.payments.on('consume', ({ product, purchase }) => {});
// Error while using
gp.payments.on('error:consume', (error) => {});
public void Consume() => GP_Payments.Consume("EXTRA_GOLD", OnConsumeSuccess, OnConsumeError);
// Successfully used
private void OnConsumeSuccess(string productIdOrTag) => Debug.Log("CONSUME: SUCCESS: " + productIdOrTag);
// Error while using
private void OnConsumeError() => Debug.Log("CONSUME: ERROR");
Spending method through promises
- JavaScript
- Unity
const result = await gp.payments.consume({ id: 17541 });
/**
* Product data
* @type {Product}
*/
result.product;
/**
* Purchase data
* @type {PlayerPurchase}
*/
result.purchase;
Not implemented
Lists of Products and Purchases
FREELists are loaded at the start of the game and are available immediately after SDK initialization.
Get the list of products:
- JavaScript
- Unity
gp.payments.products;
// Subscribe to events
private void OnEnable()
{
GP_Payments.OnFetchProducts += OnFetchProducts;
GP_Payments.OnFetchProductsError += OnFetchProductsError;
}
// Unsubscribe from events
private void OnDisable()
{
GP_Payments.OnFetchProducts -= OnFetchProducts;
GP_Payments.OnFetchProductsError -= OnFetchProductsError;
}
// Fetch the list of products through
public void FetchProducts() => GP_Payments.Fetch()
// Successfully received
private void OnFetchProducts(List<FetchProducts> products)
{
for (int i = 0; i < products.Count; i++)
{
Debug.Log("PRODUCT: ID: " + products[i].id);
Debug.Log("PRODUCT: TAG: " + products[i].tag);
Debug.Log("PRODUCT: NAME: " + products[i].name);
Debug.Log("PRODUCT: DESCRIPTION: " + products[i].description);
Debug.Log("PRODUCT: ICON: " + products[i].icon);
Debug.Log("PRODUCT: ICON SMALL: " + products[i].iconSmall);
Debug.Log("PRODUCT: PRICE: " + products[i].price);
Debug.Log("PRODUCT: CURRENCY: " + products[i].currency);
Debug.Log("PRODUCT: CURRENCY SYMBOL: " + products[i].currencySymbol);
Debug.Log("PRODUCT: IS SUBSCRIPTION: " + products[i].isSubscription);
Debug.Log("PRODUCT: PERIOD: " + products[i].period);
Debug.Log("PRODUCT: TRIAL PERIOD: " + products[i].trialPeriod);
}
}
// Errors by getting
private void OnFetchProductsError() => Debug.Log("FETCH PRODUCTS: ERROR");
Get the list of the player's purchases:
- JavaScript
- Unity
gp.payments.purchases;
// Subscribe to event
private void OnEnable()
{
GP_Payments.OnFetchPlayerPurchases += OnFetchPlayerPurchases;
}
// Unsubscribe from event
private void OnDisable()
{
GP_Payments.OnFetchPlayerPurchases -= OnFetchPlayerPurchases;
}
// Fetch the list of the player's purchases through
public void FetchPlayerPurchases() => GP_Payments.Fetch();\
// Successfully received
private void OnFetchPlayerPurchases(List<FetchPlayerPurchases> purcahses)
{
for (int i = 0; i < purcahses.Count; i++)
{
Debug.Log("PLAYER PURCHASES: PRODUCT TAG: " + purcahses[i].tag);
Debug.Log("PLAYER PURCHASES: PRODUCT ID: " + purcahses[i].productId);
Debug.Log("PLAYER PURCHASES: PAYLOAD: " + purcahses[i].payload);
Debug.Log("PLAYER PURCHASES: CREATED AT: " + purcahses[i].createdAt);
Debug.Log("PLAYER PURCHASES: EXPIRED AT: " + purcahses[i].expiredAt);
Debug.Log("PLAYER PURCHASES: GIFT: " + purcahses[i].gift);
Debug.Log("PLAYER PURCHASES: SUBSCRIBED: " + purcahses[i].subscribed);
}
}
Getting a list of products
FREE Deprecated- JavaScript
- Unity
gp.payments.fetchProducts();
GP_Payments.Fetch();
Get method via promises
- JavaScript
- Unity
const result = await gp.payments.fetchProducts();
// Answer result
const {
// Grocery list
products,
// Player shopping list
playerPurchases,
} = result;
Not implemented
Events when getting a shopping list
- JavaScript
- Unity
// Successfully received
gp.payments.on('fetchProducts', (result) => {});
// Errors by getting
gp.payments.on('error:fetchProducts', (error) => {});
//Subscribe to events
private void OnEnable()
{
GP_Payments.OnFetchProducts += OnFetchProducts;
GP_Payments.OnFetchProductsError += OnFetchProductsError;
GP_Payments.OnFetchPlayerPurchases += OnFetchPlayerPurchases;
}
//Unsubscribe from events
private void OnDisable()
{
GP_Payments.OnFetchProducts -= OnFetchProducts;
GP_Payments.OnFetchProductsError -= OnFetchProductsError;
GP_Payments.OnFetchPlayerPurchases -= OnFetchPlayerPurchases;
}
public void Fetch() => GP_Payments.Fetch();
// Successfully received
private void OnFetchProducts(List<FetchProducts> products)
{
for (int i = 0; i < products.Count; i++)
{
Debug.Log("PRODUCT: ID: " + products[i].id);
Debug.Log("PRODUCT: TAG: " + products[i].tag);
Debug.Log("PRODUCT: NAME: " + products[i].name);
Debug.Log("PRODUCT: DESCRIPTION: " + products[i].description);
Debug.Log("PRODUCT: ICON: " + products[i].icon);
Debug.Log("PRODUCT: ICON SMALL: " + products[i].iconSmall);
Debug.Log("PRODUCT: PRICE: " + products[i].price);
Debug.Log("PRODUCT: CURRENCY: " + products[i].currency);
Debug.Log("PRODUCT: CURRENCY SYMBOL: " + products[i].currencySymbol);
Debug.Log("PRODUCT: IS SUBSCRIPTION: " + products[i].isSubscription);
Debug.Log("PRODUCT: PERIOD: " + products[i].period);
Debug.Log("PRODUCT: TRIAL PERIOD: " + products[i].trialPeriod);
}
}
// Errors by getting
private void OnFetchProductsError() => Debug.Log("FETCH PRODUCTS: ERROR");
private void OnFetchPlayerPurchases(List<FetchPlayerPurchases> purcahses)
{
for (int i = 0; i < purcahses.Count; i++)
{
Debug.Log("PLAYER PURCHASES: PRODUCT TAG: " + purcahses[i].tag);
Debug.Log("PLAYER PURCHASES: PRODUCT ID: " + purcahses[i].productId);
Debug.Log("PLAYER PURCHASES: PAYLOAD: " + purcahses[i].payload);
Debug.Log("PLAYER PURCHASES: CREATED AT: " + purcahses[i].createdAt);
Debug.Log("PLAYER PURCHASES: EXPIRED AT: " + purcahses[i].expiredAt);
Debug.Log("PLAYER PURCHASES: GIFT: " + purcahses[i].gift);
Debug.Log("PLAYER PURCHASES: SUBSCRIBED: " + purcahses[i].subscribed);
}
}
Purchase fields
Field | Type | Description | Example |
---|---|---|---|
id | number | Purchase ID | 115 |
tag | string | Optional tag to help with selection. You can use it instead of ID | VIP |
name | string | Name translated into the user's language | VIP Status |
description | string | Description translated into the user's language | No ads, reward x2 |
icon | string | Link to icon size 256x256 | Link example |
iconSmall | string | Link to icon size 64x64 | Link example |
price | number | Purchase price | 199 |
currency | string | Currency code | YAN OK VOTE RUB GP |
currencySymbol | string | Currency symbol (translated into the user's language and formatted if needed) | Voice, Voices |
isSubscription | boolean | Purchase is a subscription | default false |
period | number | Subscription duration in days | 7 , 30 , 90 |
trialPeriod | number | The duration of the trial period in days (during this time, the user can cancel the subscription without debiting funds) | 0 , 3 , 7 , 30 |
Player Purchase Fields
Field | Type | Description | Example |
---|---|---|---|
productId | number | Purchase ID | 115 |
payload | Object | Data from the purchase on the platform (for example, order ID or token) | {"order_id": 213} |
createdAt | string | Date of purchase, format ISO 8601 UTC | 2022-12-01T04:52:26+0000 |
gift | boolean | The purchase was a gift from the game developer | false |
subscribed | boolean | Is the subscription for this purchase active? | true |
expiredAt | string | Subscription end date, format ISO 8601 UTC | 2022-12-01T04:52:26+0000 |
Error codes
Error | Error Description |
---|---|
player_not_found | Player not found |
empty_id_or_tag | Passed empty ID or purchase tag |
product_not_found | Purchase with this ID or tag was not found |
purchases_not_alloved_on_platform | Purchases are not available on this site |
undefined | Unexpected error (see console) |
Stay in Touch
Other documents of this chapter available Here. To get started, welcome to the Tutorials chapter.
GamePush Community Telegram
: @gs_community.
For your suggestions e-mail
: [email protected]
We Wish you Success!