Social actions
Overview
We suggest using social actions for audience multiplication — share, post and invite friends to the game. Platforms such as VK and OK allow you to use the tools built into the platform. The rest of the platforms will imitate the functionality through the usual link sharing with the ability to add a comment and sometimes pictures.
The list of supported social networks and messengers for sharing:
- Telegram
- Vkontakte
- Odnoklassniki
- Viber
For better parsing of games by messengers and social networks, we recommend placing Open Graph meta tags in the game. And also fill in the title and description. This is useful in terms of SEO optimization. An example of placing meta tags is shown below:
// Fill in the basic information about the game
<title>My awesome game 2</title>
<meta name="og:title" content="My awesome game 2">
<meta name="description" content="There are not enough words to describe the awesomeness of the game">
<meta name="og:description" content="There are not enough words to describe the awesomeness of the game">
<meta name="og:image" content="/img/ogimage.png">
Supported platforms
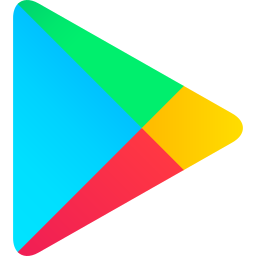
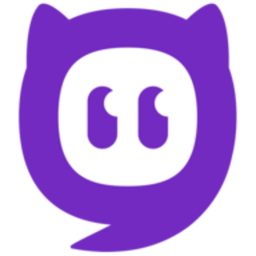
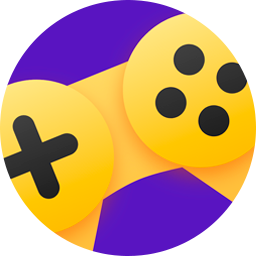
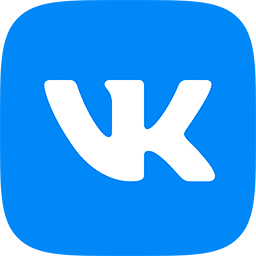
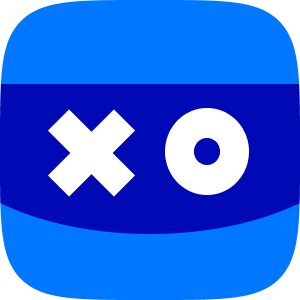
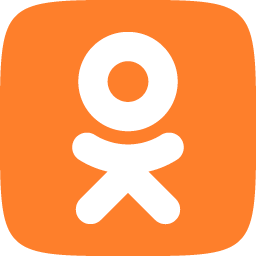
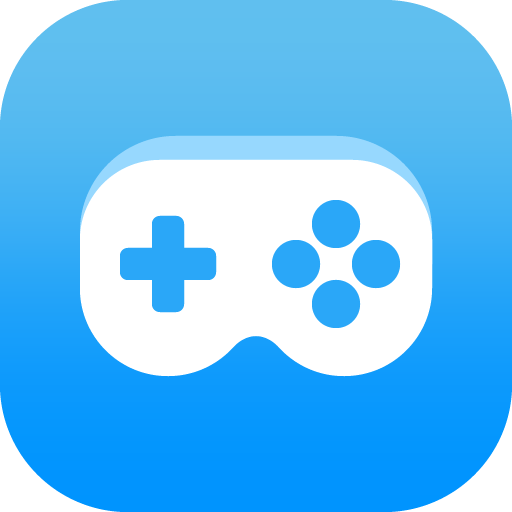
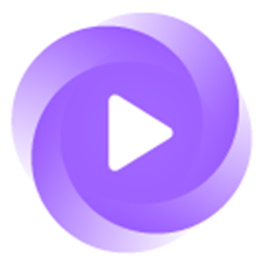
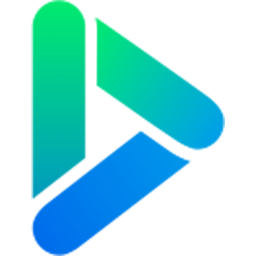
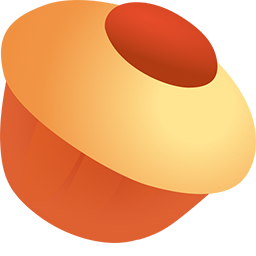
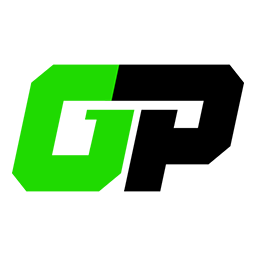
Platforms without support
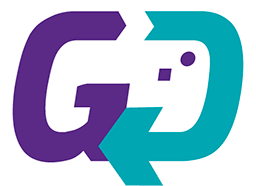
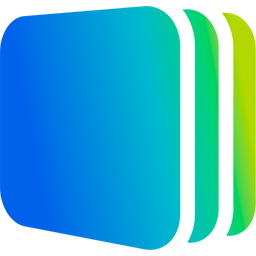
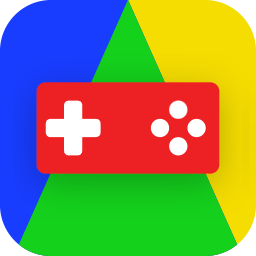
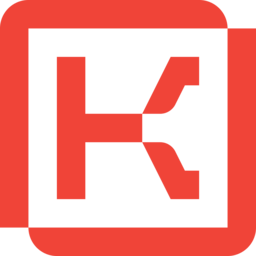
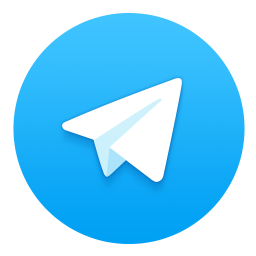
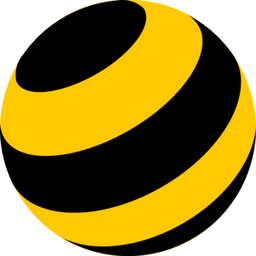
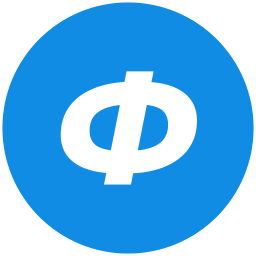
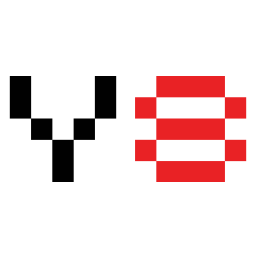
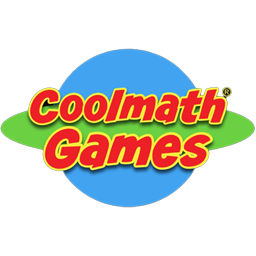
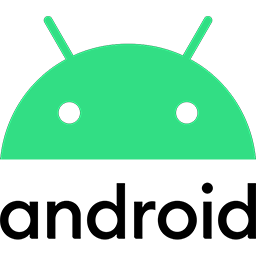
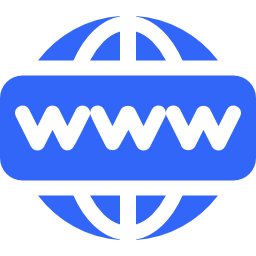
To check that sharing is allowed and supported on the platform:
- JavaScript
- Unity
// Is sharing supported
gp.socials.isSupportsShare;
// Is sharing supported
GP_Socials.IsSupportsShare();
Share
FREEBy default, when calling the share
method, you can share a link to the game. We will determine the link ourselves based on the platform and insert the necessary text. The text is taken from the project name in the control panel or from the og:title
meta tag or simply from the title
tag.
Platforms with native functionality
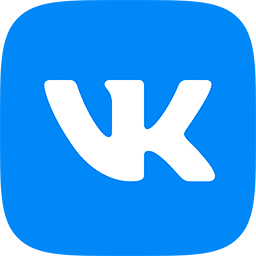
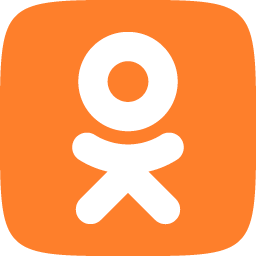
Platforms without support
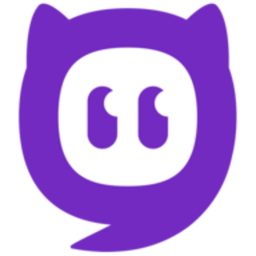
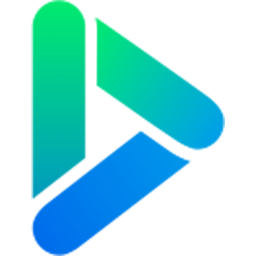
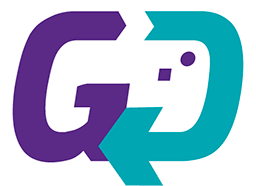
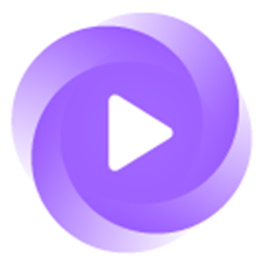
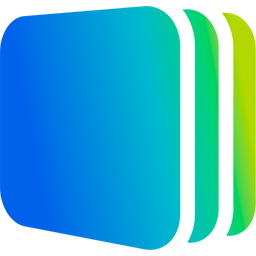
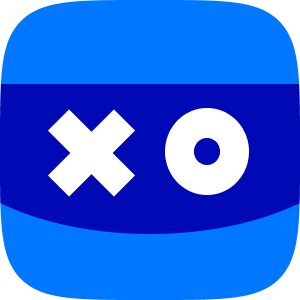
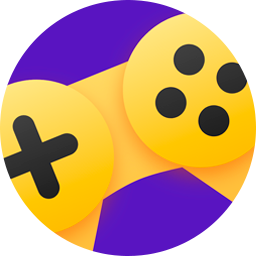
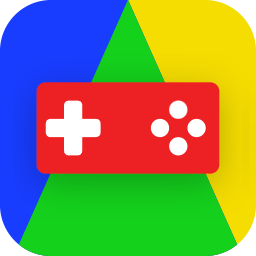
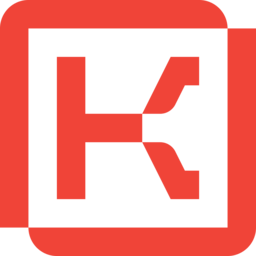
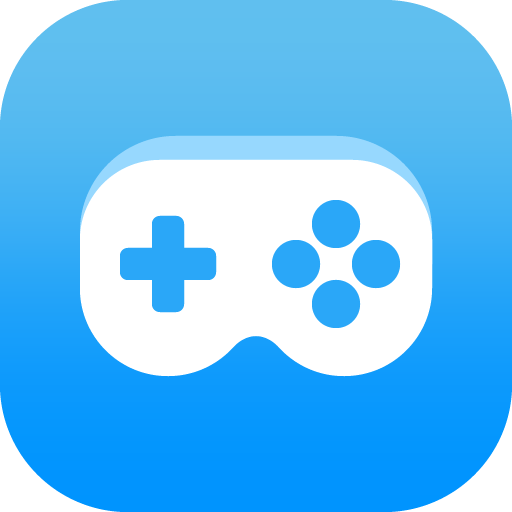
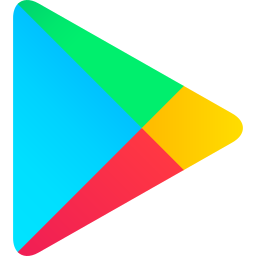
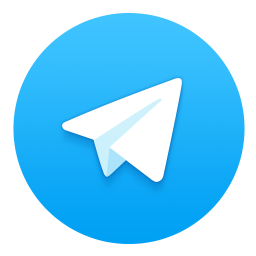
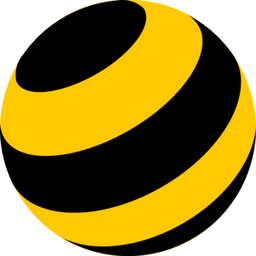
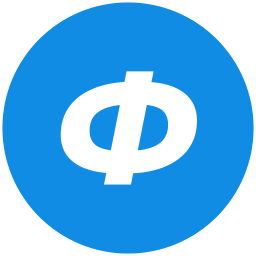
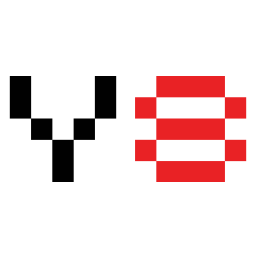
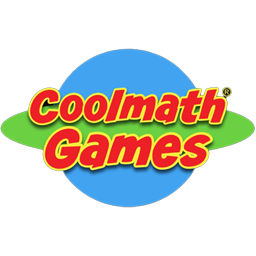
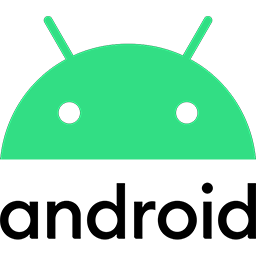
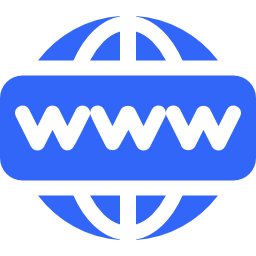
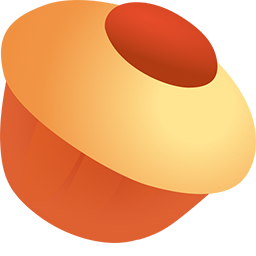
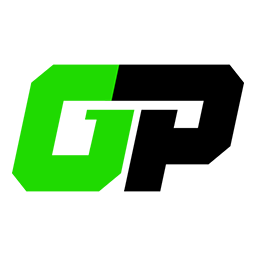
- JavaScript
- Unity
// Is native sharing supported
gp.socials.isSupportsNativeShare;
// Is native sharing supported
GP_Socials.IsSupportsNativeShare();
In platforms without native support for this method, there will be used an overlay with an offer to share on social networks and messengers.
- JavaScript
- Unity
gp.socials.share();
GP_Socials.Share();
Share with free text adding:
- JavaScript
- Unity
gp.socials.share({
text: `I tapped the square 73 times in 5 seconds!
Now I am number 23 in "Fastest Fingers" list.
Can you beat out me?`,
});
GP_Socials.Share(text = 'I tapped the square 73 times in 5 seconds!
Now I am number 23 in "Fastest Fingers" list.
Can you beat out me?');
Share with an arbitrary link adding:
- JavaScript
- Unity
gp.socials.share({
url: `${gp.app.url}?invitedBy=${gp.player.id}`,
});
GP_Socials.Share(url = GP_App.Url());
Share with an optional picture adding:
- JavaScript
- Unity
gp.socials.share({
image: 'https://gamepush.com/img/ogimage.png',
});
GP_Socials.Share(image = "https://gamepush.com/img/ogimage.png");
Support for images through native methods of social networks is implemented only in Vkontakte through the attachment format photo123456_123456
and Odnoklassniki through the attachment format 123456
, or URL of images uploaded through GamePush to the platform (gp.images.upload()
) for the rest, the image parameter will be ignored, only url and text are available.
Fully customizable sharing:
- JavaScript
- Unity
gp.socials.share({
text: 'Join me in the game "My awesome game 2"',
url: gp.app.url,
image: 'https://gamepush.com/img/ogimage.png',
});
GP_Socials.Share(
text = 'Присоединяйся ко мне в игре "My awesome game 2"',
url = GP_App.Url(),
image = 'https://gamepush.com/img/ogimage.png'
);
You can subscribe to on share event:
- JavaScript
- Unity
gp.socials.on('share', (success) => {
// success = true if successful share
});
//Subscribe to event
private void OnEnable()
{
GP_Socials.OnShare += OnShare;
}
//Unsubscribe from event
private void OnDisable()
{
GP_Socials.OnShare -= OnShare;
}
// success = true if successful share
private void OnShare(bool success) => ConsoleUI.Instance.Log("SOCIALS: SHARE: " + success);
Post
FREEAs opposed to share method, the post method involves posting to the player’s friends news feed.
Platforms with native functionality
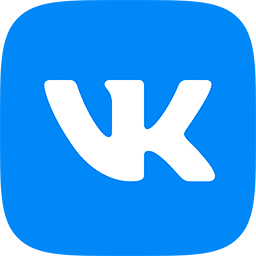
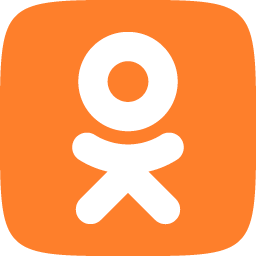
Platforms without support
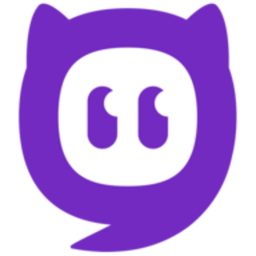
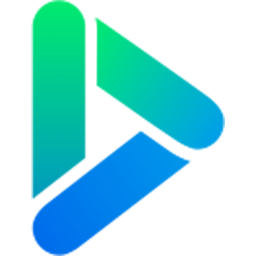
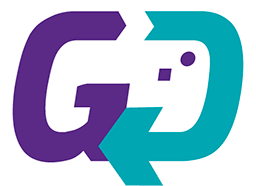
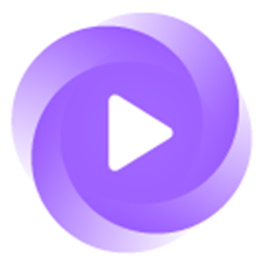
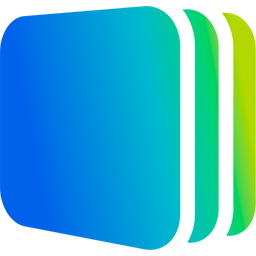
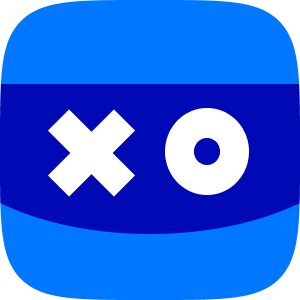
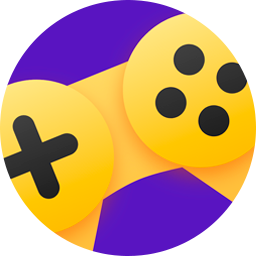
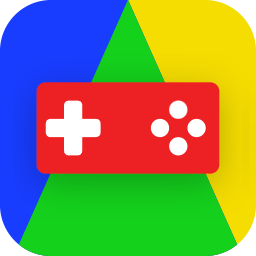
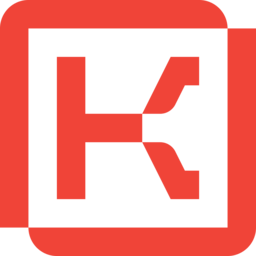
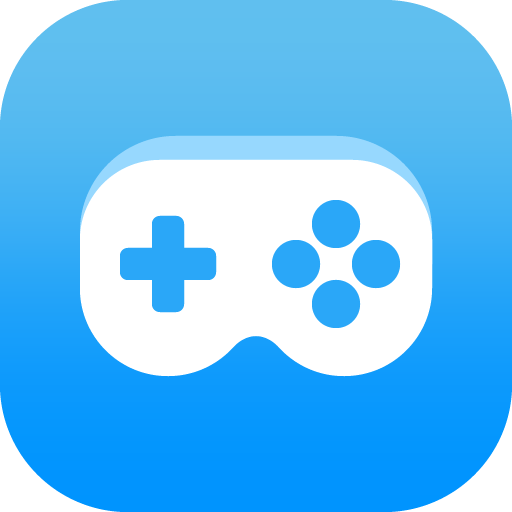
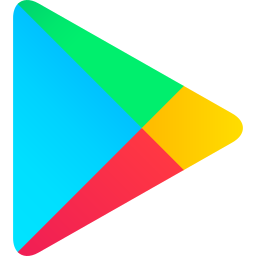
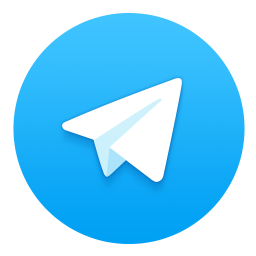
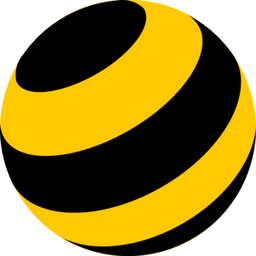
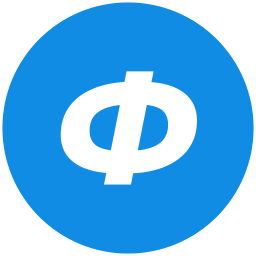
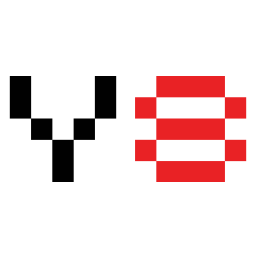
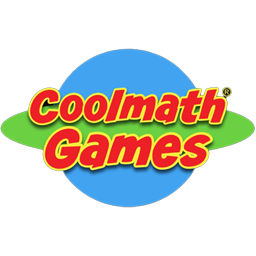
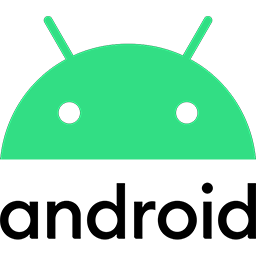
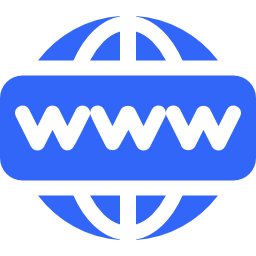
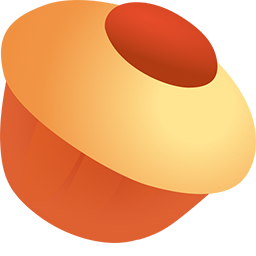
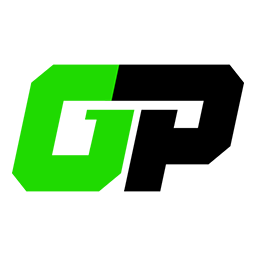
- JavaScript
- Unity
// Is native posting supported
gp.socials.isSupportsNativePosts;
// Is native posting supported
GP_Socials.IsSupportsNativePosts();
On the platforms without native supporting for this method, there will be used an overlay with an offer to share, as in the case of the share
method.
The usage logic duplicates the share
method.
- JavaScript
- Unity
gp.socials.post();
GP_Socials.Post();
Fully customizable post:
- JavaScript
- Unity
gp.socials.post({
text: 'Join me in the game "My awesome game 2"',
url: gp.app.url,
image: 'https://gamepush.com/img/ogimage.png',
});
GP_Socials.Share(
text = 'Присоединяйся ко мне в игре "My awesome game 2"',
url = GP_App.Url(),
image = 'https://gamepush.com/img/ogimage.png'
);
You can subscribe to on post event:
- JavaScript
- Unity
gp.socials.on('post', (success) => {
// success = true if successful post
});
//Subscribe to event
private void OnEnable()
{
GP_Socials.OnPost += OnPost;
}
//Unsubscribe from event
private void OnDisable()
{
GP_Socials.OnPost -= OnPost;
}
// success = true, если успешно выполнено действие "опубликовать"
private void OnPost(bool success) => ConsoleUI.Instance.Log("SOCIALS: ON POST: " + success);
Invite friends
FREEShows the player a friends list to whom you can send the invitation.
Platforms with native functionality
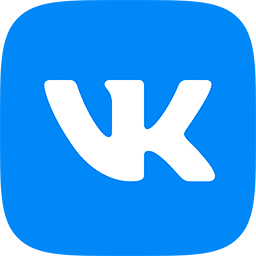
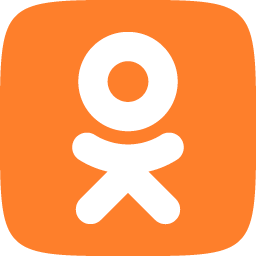
Platforms without support
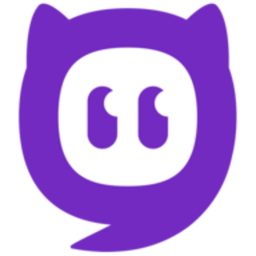
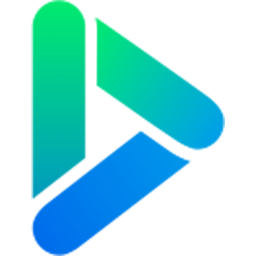
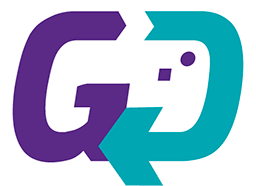
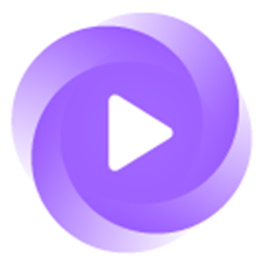
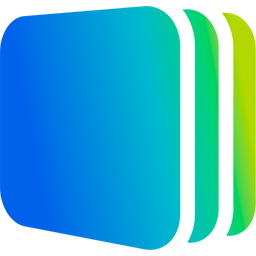
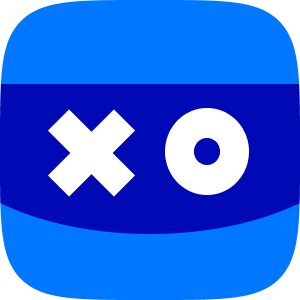
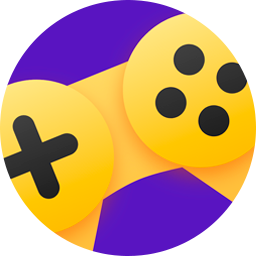
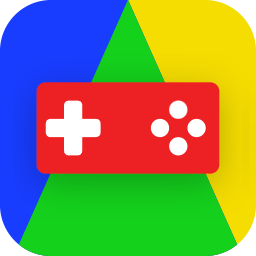
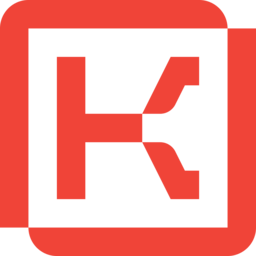
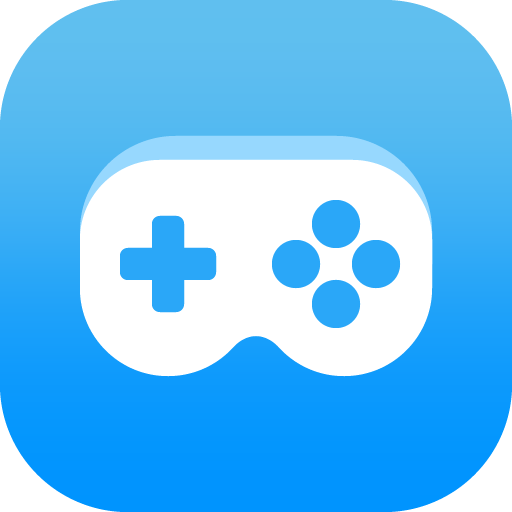
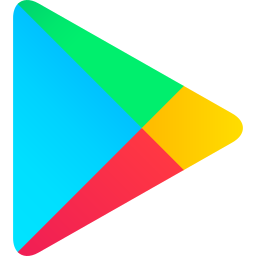
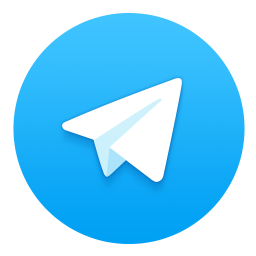
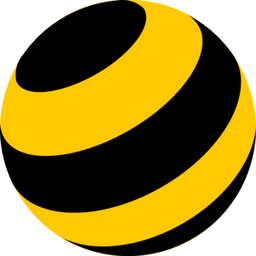
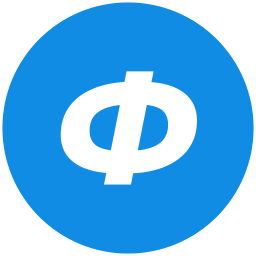
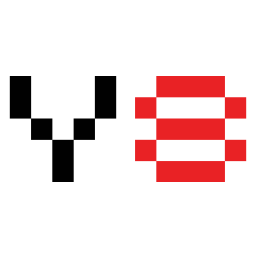
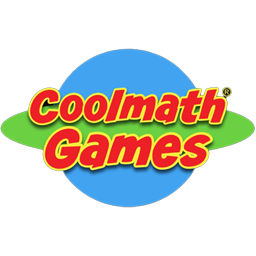
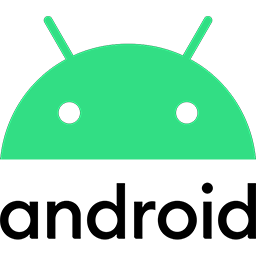
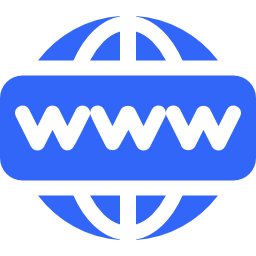
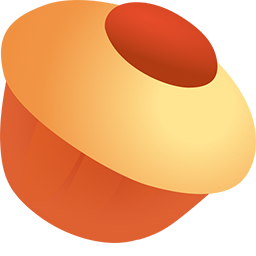
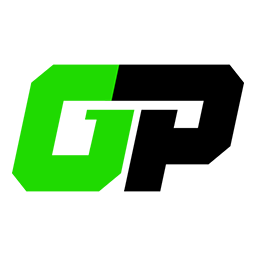
- JavaScript
- Unity
// Are native invites supported
gp.socials.isSupportsNativeInvite;
// Are native invites supported
GP_Socials.IsSupportsNativeInvite();
On the platform without native supporting of this method, there will be used an overlay with the offer to invite friends, as in the case of share
and post
methods.
The usage logic duplicates the share
method.
- JavaScript
- Unity
gp.socials.invite();
GP_Socials.Invite();
Customizable text for some platforms:
- JavaScript
- Unity
gp.socials.invite({
text: 'Join me in the game "My awesome game 2"',
});
GP_Socials.Invite(
text = 'Join me in the game "My awesome game 2"',
url = GP_App.Url(),
image = 'https://gamepush.com/img/ogimage.png'
);
You can subscribe to on invite event:
- JavaScript
- Unity
gp.socials.on('invite', (success) => {
// success = true if successful invite
});
//Subscribe to event
private void OnEnable()
{
GP_Socials.OnInvite += OnInvite;
}
//Unsubscribe from event
private void OnDisable()
{
GP_Socials.OnInvite -= OnInvite;
}
// success = true if successful invite
private void OnInvite(bool success) => ConsoleUI.Instance.Log("SOCIALS: ON INVITE: " + success);
The method accepts the same object as in the case of share
and post
, but today only text
is used.
Join community
FREEShows the player an overlay with the opportunity to join the community (if the platform allows) or opens a link to the community in a new tab.
Supported platforms
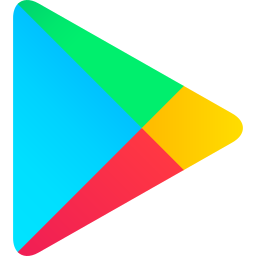
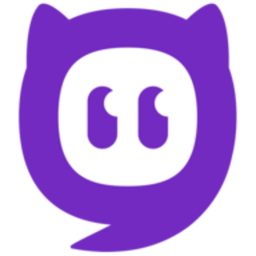
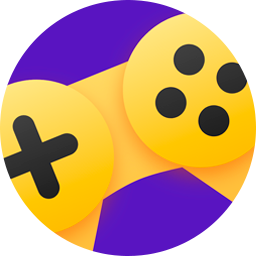
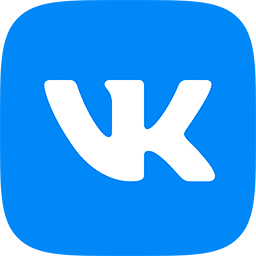
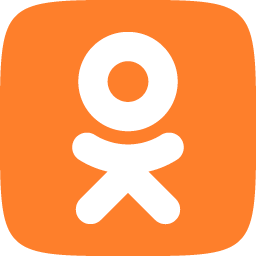
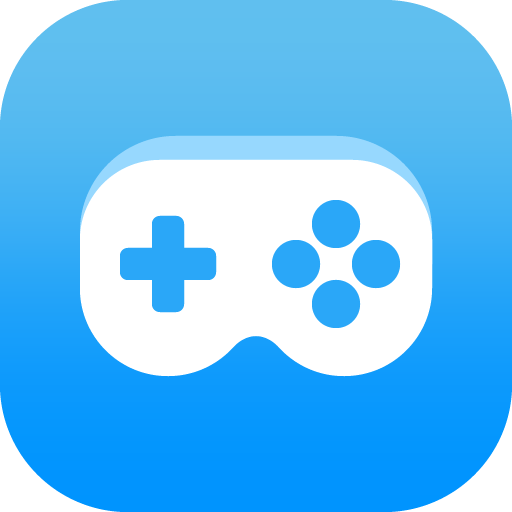
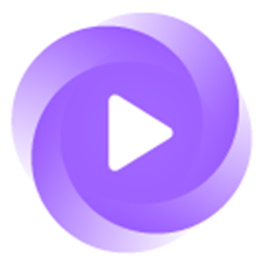
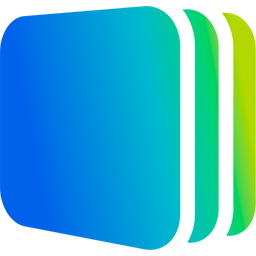
Platforms without support
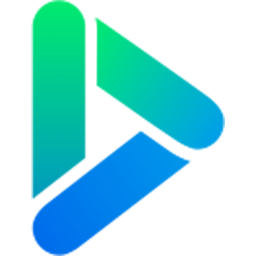
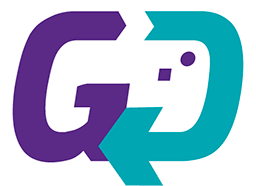
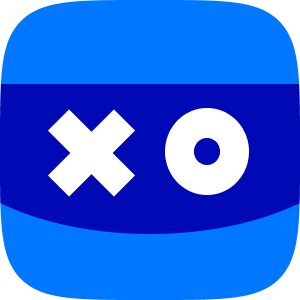
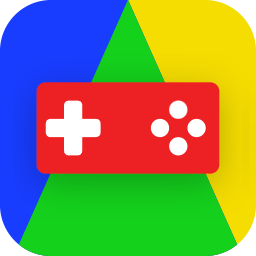
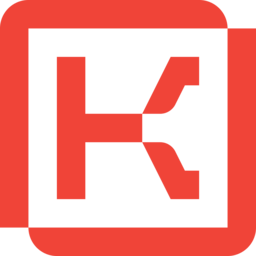
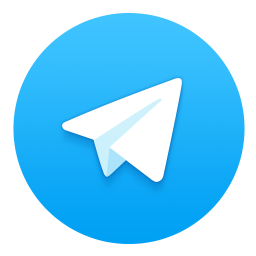
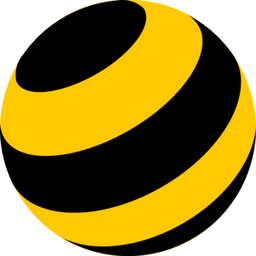
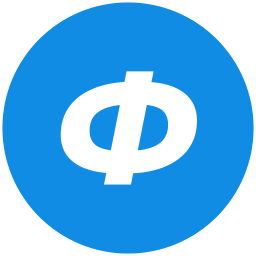
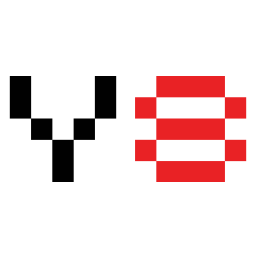
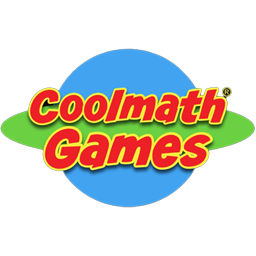
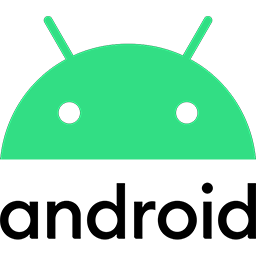
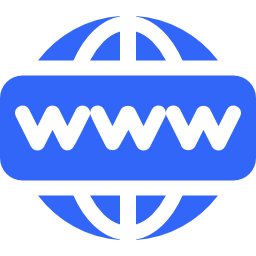
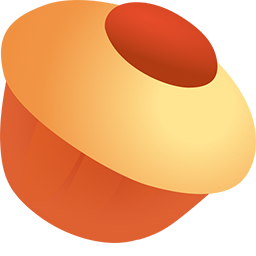
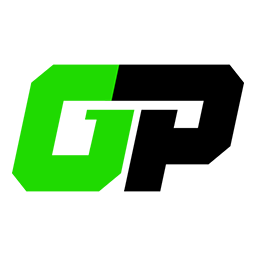
- JavaScript
- Unity
// check is community join is available on platform
gp.socials.canJoinCommunity;
// check is community join is available on platform
GP_Socials.CanJoinCommunity();
Platforms with native functionality
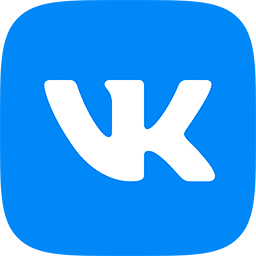
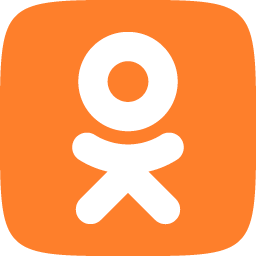
Platforms without support
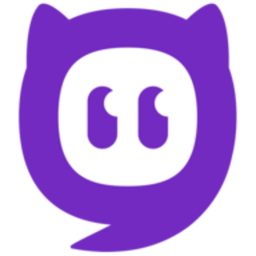
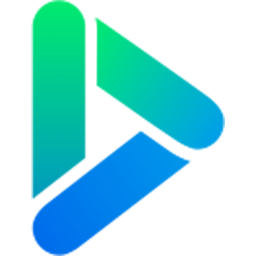
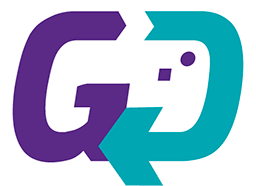
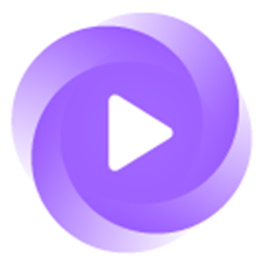
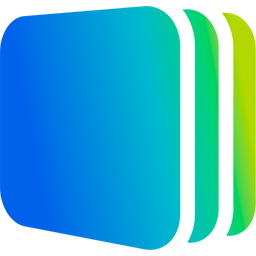
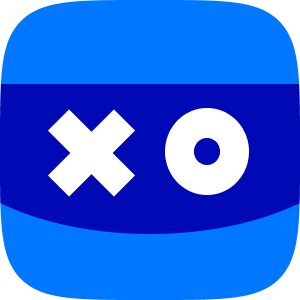
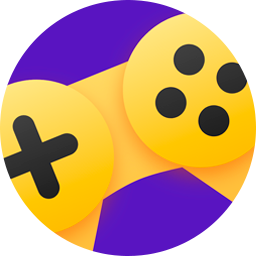
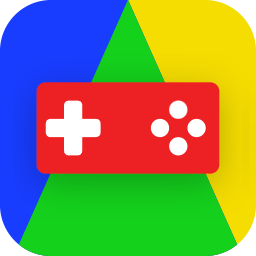
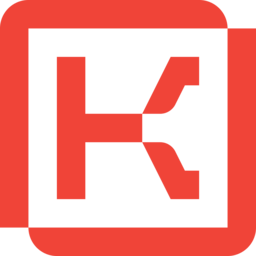
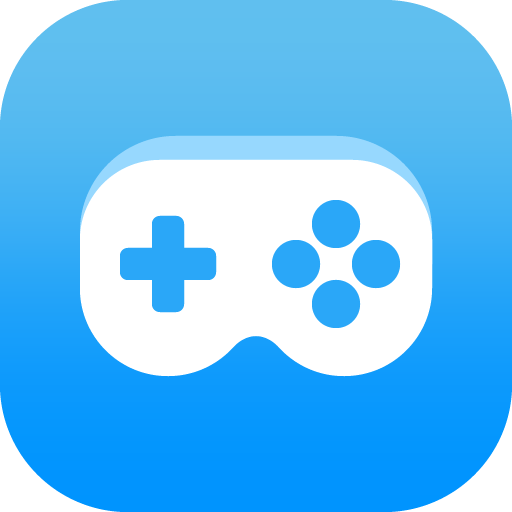
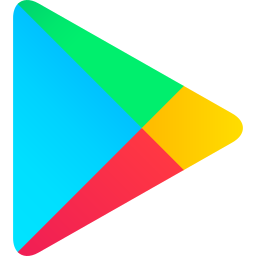
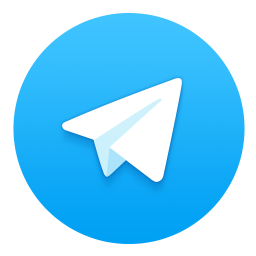
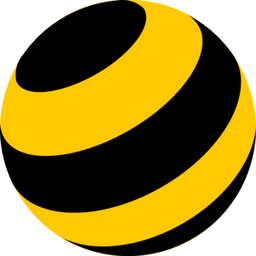
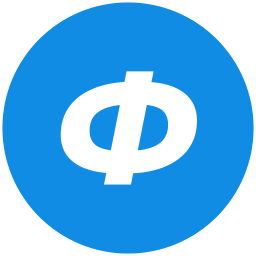
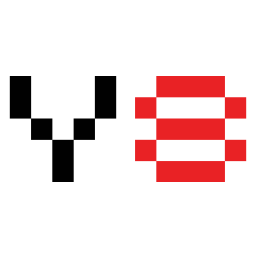
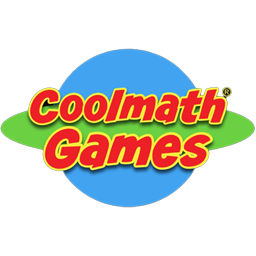
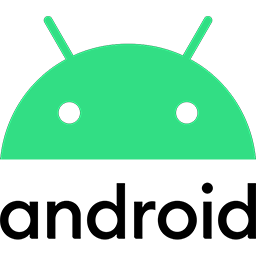
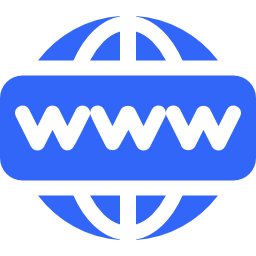
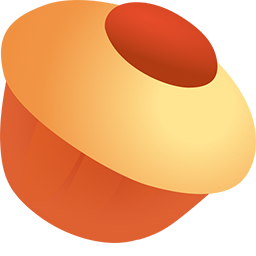
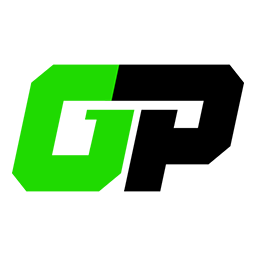
- JavaScript
- Unity
// check is native community join is supported
gp.socials.isSupportsNativeCommunityJoin;
// check is native community join is supported
GP_Socials.IsSupportsNativeCommunityJoin();
Join community:
- JavaScript
- Unity
gp.socials.joinCommunity();
GP_Socials.JoinCommunity();
You can subscribe to on join community event:
- JavaScript
- Unity
gp.socials.on('joinCommunity', (success) => {
// success = true if successful join community
});
//Subscribe to event
private void OnEnable()
{
GP_Socials.OnJoinCommunity += OnJoinCommunity;
}
//Unsubscribe from event
private void OnDisable()
{
GP_Socials.OnJoinCommunity -= OnJoinCommunity;
}
// success = true if successful join community
private void OnJoinCommunity(bool success) => ConsoleUI.Instance.Log("SOCIALS: ON JOIN COMMUNITY: " + success);
Working with parameters for sharing
You may need to create a link to invite a player that contains the inviter's ID and a gift for the invited player. To do this, you need to add these parameters to the game link on the platform. All platforms require different ways of forming URLs. We have combined the creation of links into one method. When you follow this link, all previously passed parameters are available to you.
Create a sharing link
FREE- JavaScript
- Unity
const shareUrl = gp.socials.makeShareUrl({
// any of your parameters
fromId: gp.player.id,
gift: 'GOLD_SWORD_X5',
});
// https://vk.com/app7869399#eyJmcm9tSWQiOjE4MDcwMTksImdpZnQiOiJHT0xEX1NXT1JEX1g1In0=
public string MakeShareLink(string content)
{
return GP_Socials.MakeShareLink(content);
}
Read parameters when following the link
FREE- JavaScript
- Unity
// any of your parameters specified in the link
const fromId = gp.socials.getShareParam('fromId'); // 123456
const gift = gp.socials.getShareParam('gift'); // "GOLD_SWORD_X5"
// ID of the player who shared the link
int sharePlayerID = GP_Socials.GetSharePlayerID();
// Content in the link
string shareContent = GP_Socials.GetShareContent();
Stay in Touch
Other documents of this chapter available Here. To get started, welcome to the Tutorials chapter.
GamePush Community Telegram
: @gs_community.
For your suggestions e-mail
: [email protected]
We Wish you Success!